环境
- mac book pro
- unity 2022.3.7f
1 2 3 4
| // 依赖包 "com.unity.entities.graphics": "1.0.10", "com.unity.feature.development": "1.0.1", "com.unity.render-pipelines.universal": "14.0.8",
|
实战
- 新建一个场景
scene_cubes_subscene
,创建一个空对象,添加组件SpawnerAuthoring
- 新建一个场景
scene_cubes
,创建一个空对象,添加SubScene
指向子场景scene_cubes_subscene
- 运行场景
scene_cubes
SpawnerAuthoring.cs1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| using Unity.Entities; using UnityEngine;
namespace Scenes.Cubes { public class SpawnerAuthoring : MonoBehaviour { public GameObject GoPrefab; public int XNum = 10; public int ZNum = 10; class Baker : Baker<SpawnerAuthoring> { public override void Bake(SpawnerAuthoring authoring) { var entity = GetEntity(TransformUsageFlags.None); Debug.Log("1:" + entity.Index); AddComponent(entity, new Spawner { GoPrefab = GetEntity(authoring.GoPrefab, TransformUsageFlags.Dynamic), XNum = authoring.XNum, ZNum = authoring.ZNum, }); } } } public struct Spawner : IComponentData { public Entity GoPrefab; public int XNum; public int ZNum; } }
|
SpawnSystem.cs1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66
| using Unity.Burst; using Unity.Collections; using Unity.Entities; using Unity.Mathematics; using Unity.Transforms; using UnityEngine;
namespace Scenes.Cubes { public partial struct SpawnSystem : ISystem { private bool mIsCreate; [BurstCompile] public void OnCreate(ref SystemState state) { state.RequireForUpdate<Spawner>(); } [BurstCompile] public void OnUpdate(ref SystemState state) { if (mIsCreate) { return; }
mIsCreate = true; CreateEntities(ref state); }
private void CreateEntities(ref SystemState state) { Spawner spawner = SystemAPI.GetSingleton<Spawner>(); int entityNum = spawner.XNum * spawner.ZNum; var instances = state.EntityManager.Instantiate(spawner.GoPrefab, entityNum, Allocator.Temp);
int index = 0; for (int x = 0; x < spawner.XNum; x++) { for (int z = 0; z < spawner.ZNum; z++) { Entity tmpEntity = instances[index]; state.EntityManager.AddComponent<Rotate>(tmpEntity); var rot = SystemAPI.GetComponentRW<Rotate>(tmpEntity); rot.ValueRW.RotateSpeed = UnityEngine.Random.Range(5, 15); state.EntityManager.AddComponent<Movement>(tmpEntity); var move = SystemAPI.GetComponentRW<Movement>(tmpEntity); move.ValueRW.MoveSpeed = UnityEngine.Random.Range(1, 5); var transform = SystemAPI.GetComponentRW<LocalTransform>(tmpEntity); float3 pos = new float3(x - spawner.XNum / 2, noise.cnoise(new float2(x, z)), z - spawner.ZNum / 2); transform.ValueRW.Position = pos;
index++; } } } } }
|
RotateSystem.cs1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| using Unity.Burst; using Unity.Entities; using Unity.Mathematics; using Unity.Transforms; using UnityEngine;
namespace Scenes.Cubes { public partial struct RotateSystem : ISystem { [BurstCompile] public void OnCreate(ref SystemState state) { state.RequireForUpdate<Rotate>(); }
[BurstCompile] public void OnUpdate(ref SystemState state) { foreach (var (transform, rotate) in SystemAPI.Query<RefRW<LocalTransform>, RefRO<Rotate>>()) { transform.ValueRW.Rotation = math.mul(math.normalize(transform.ValueRW.Rotation), quaternion.AxisAngle(math.up(), rotate.ValueRO.RotateSpeed * Time.deltaTime)); } } } public struct Rotate : IComponentData { public float RotateSpeed; } }
|
MoveSystem.cs1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| using Unity.Burst; using Unity.Entities; using Unity.Mathematics; using Unity.Transforms; using UnityEngine;
namespace Scenes.Cubes { public partial struct MoveSystem : ISystem { [BurstCompile] public void OnCreate(ref SystemState state) { state.RequireForUpdate<Movement>(); }
[BurstCompile] public void OnUpdate(ref SystemState state) { foreach (var (transform, movement) in SystemAPI.Query<RefRW<LocalTransform>, RefRW<Movement>>()) { float3 pos = transform.ValueRW.Position; pos.y += movement.ValueRW.MoveSpeed * Time.deltaTime; if (pos.y > 4) { movement.ValueRW.MoveSpeed = -Mathf.Abs(movement.ValueRW.MoveSpeed); } else if (pos.y < -1) { movement.ValueRW.MoveSpeed = Mathf.Abs(movement.ValueRW.MoveSpeed); }
transform.ValueRW.Position = pos; } } } public struct Movement : IComponentData { public float MoveSpeed; } }
|
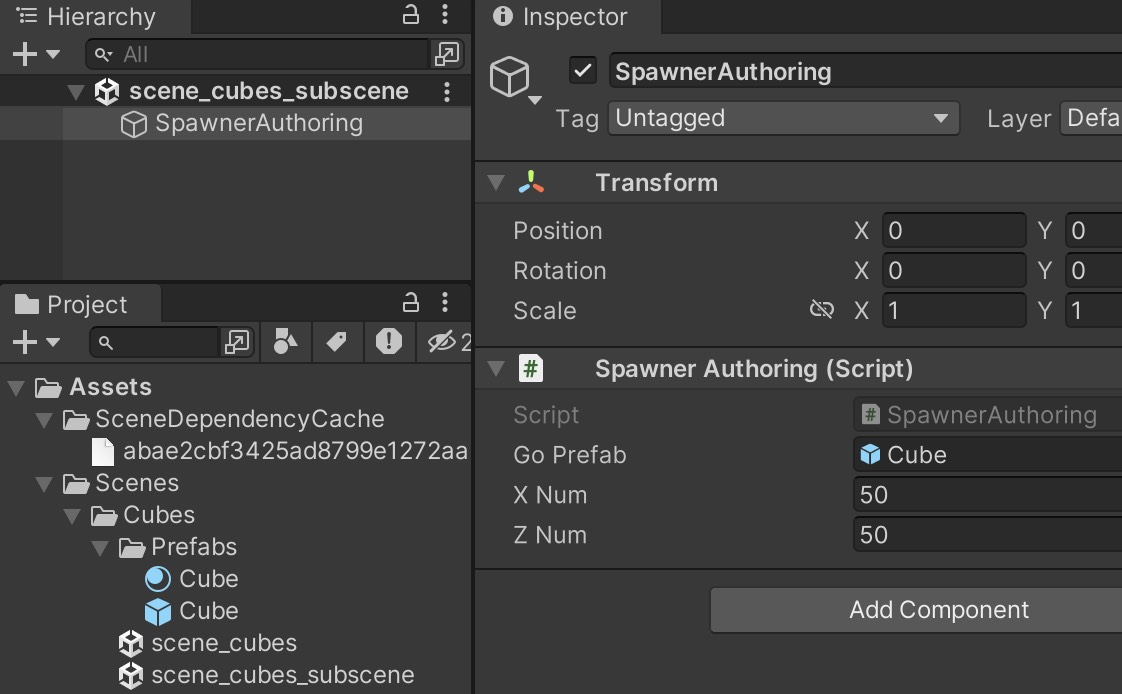
scene_cubes_subscene
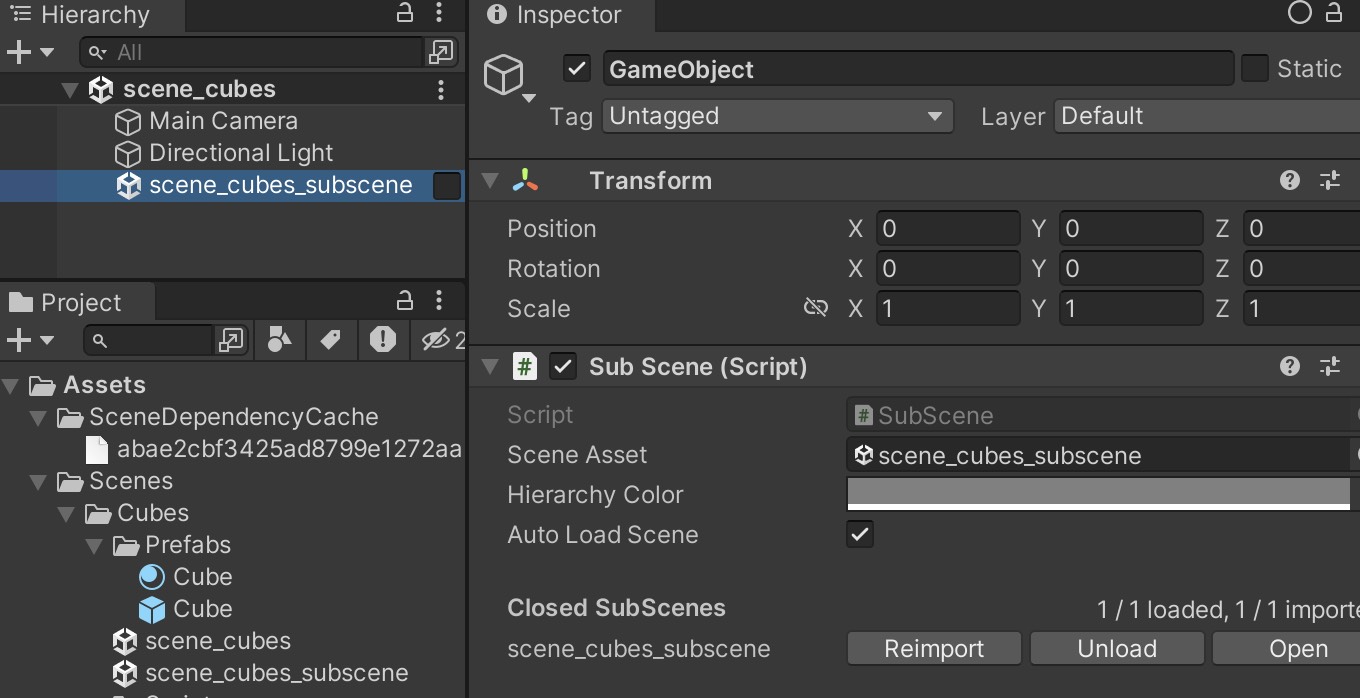
scene_cubes
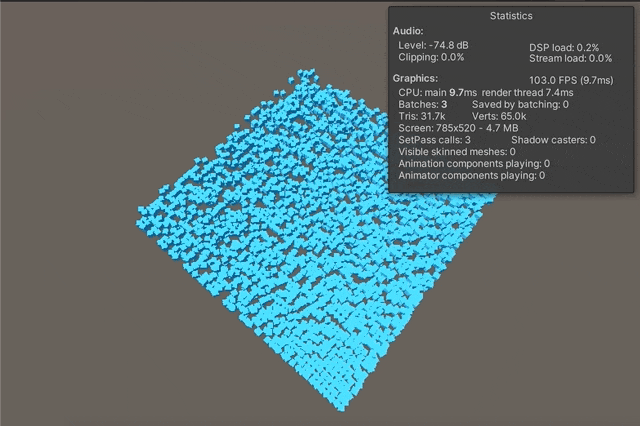
运行效果图
链接
[Entity1.0.14 api] https://docs.unity3d.com/Packages/com.unity.entities@1.0/manual/ecs-workflow-scene.html
[代码地址] https://gitee.com/hahafox_0/unity-tutorial-ecs2022.git